mirror of
https://github.com/schollz/cowyo.git
synced 2023-08-10 21:13:00 +03:00
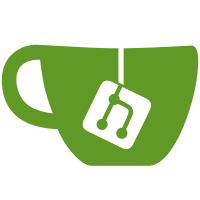
Former-commit-id: d95a6e65969ae44a87ca5f705e98973ca8b6d499 [formerly 41145c54c8b0d5eccfca8f11f7b6d1f5bbec5cf4] [formerly 74f59fa690178790d3e3ca03a16a35e7a07c2c02 [formerly a20420cdb793e7aec8c3a271cbbf45fcee039757 [formerly8c52173484
]]] Former-commit-id: a8dcf6097d6e961d73f3de4abb55b1138119eaf5 [formerly 30ca5b6878485711f33f75143c11c62ef00fc354] Former-commit-id: a297a38187e5ec94f12146429a76268d9d71d6d1 Former-commit-id:2a83b3dd53
61 lines
1.7 KiB
JavaScript
61 lines
1.7 KiB
JavaScript
$(document).ready(function() {
|
|
var isTyping = false;
|
|
var typingTimer; //timer identifier
|
|
var updateInterval;
|
|
var doneTypingInterval = 100; //time in ms, 5 second for example
|
|
|
|
//on keyup, start the countdown
|
|
$('#emit').keyup(function() {
|
|
clearTimeout(typingTimer);
|
|
clearInterval(updateInterval);
|
|
typingTimer = setTimeout(doneTyping, doneTypingInterval);
|
|
});
|
|
|
|
//on keydown, clear the countdown
|
|
$('#emit').keydown(function() {
|
|
clearTimeout(typingTimer);
|
|
clearInterval(updateInterval);
|
|
document.title = "[UNSAVED] " + title_name;
|
|
});
|
|
|
|
//user is "finished typing," do something
|
|
function doneTyping() {
|
|
payload = JSON.stringify({ TextData: $('#emit_data').val(), Title: title_name, UpdateServer: true, UpdateClient: false })
|
|
send(payload)
|
|
console.log("Done typing")
|
|
updateInterval = setInterval(updateText, doneTypingInterval);
|
|
document.title = "[SAVED] " + title_name;
|
|
}
|
|
|
|
function updateText() {
|
|
console.log("Getting server's latest copy")
|
|
payload = JSON.stringify({ TextData: $('#emit_data').val(), Title: title_name, UpdateServer: false, UpdateClient: true })
|
|
send(payload)
|
|
}
|
|
|
|
// websockets
|
|
url = 'ws://'+external_ip+'/ws';
|
|
c = new WebSocket(url);
|
|
|
|
send = function(data){
|
|
console.log("Sending: " + data)
|
|
c.send(data)
|
|
}
|
|
|
|
c.onmessage = function(msg){
|
|
console.log(msg)
|
|
data = JSON.parse(msg.data);
|
|
if (data.UpdateClient == true) {
|
|
console.log("Updating...")
|
|
$('#emit_data').val(data.TextData)
|
|
document.title = "[LOADED] " + title_name;
|
|
}
|
|
console.log(data)
|
|
}
|
|
|
|
c.onopen = function(){
|
|
updateText();
|
|
updateInterval = setInterval(updateText, doneTypingInterval);
|
|
}
|
|
});
|