mirror of
https://github.com/askn/faker.git
synced 2023-08-10 21:13:01 +03:00
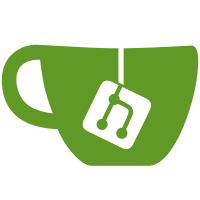
All the random functions are now passed through Faker.rng, a random number generator that is created by default, but can also be set with `Faker.seed`. The tests use all the examples in the README to ensure that everything has been seeded properly. The only module not updated is Finance, but that's because the file itself did not seem to compile, and there weren't any examples of using it in the README.
67 lines
2.1 KiB
Crystal
67 lines
2.1 KiB
Crystal
require "./spec_helper"
|
|
|
|
describe Faker::Commerce do
|
|
it "color" do
|
|
Faker::Commerce.color.match(/[a-z]+\.?/).should_not eq nil
|
|
end
|
|
|
|
it "department" do
|
|
Faker::Commerce.department.match(/[A-Z][a-z]+\.?/).should_not eq nil
|
|
end
|
|
|
|
it "single_department_should_not_contain_separators" do
|
|
Faker::Commerce.department(1).match(/\A[A-Za-z]+\z/).should_not eq nil
|
|
end
|
|
|
|
it "department_should_have_ampersand_as_default_separator" do
|
|
Faker::Commerce.department(2, true).scan(" & ").should_not eq nil
|
|
end
|
|
|
|
it "department_should_have_exact_number_of_categories_when_fixed_amount" do
|
|
Faker::Commerce.department(10, true).match(/\A([A-Za-z]+, ){8}[A-Za-z]+ & [A-Za-z]+\z/).should_not eq nil
|
|
end
|
|
|
|
it "department_should_never_exceed_the_max_number_of_categories_when_random_amount" do
|
|
100.times do
|
|
Faker::Commerce.department(6).match(/\A([A-Za-z]+(, | & )){0,5}[A-Za-z]+\z/).should_not eq nil
|
|
end
|
|
end
|
|
|
|
it "department_should_have_no_duplicate_categories" do
|
|
department = Faker::Commerce.department(10, true)
|
|
|
|
departments = department.split(/[,& ]+/)
|
|
departments.should eq departments.uniq
|
|
end
|
|
|
|
it "product_name" do
|
|
Faker::Commerce.product_name.match(/[A-Z][a-z]+\.?/).should_not eq nil
|
|
end
|
|
|
|
it "material" do
|
|
Faker::Commerce.material.match(/[A-Z][a-z]+\.?/).should_not eq nil
|
|
end
|
|
|
|
it "price" do
|
|
(0 <= Faker::Commerce.price <= 100).should be_true
|
|
(5 <= Faker::Commerce.price(5..6) <= 6).should be_true
|
|
(990 <= Faker::Commerce.price(990...1000) <= 1000).should be_true
|
|
Faker::Commerce.price(5..6).is_a?(Float).should be_true
|
|
end
|
|
|
|
it "price_is_float" do
|
|
Faker::Commerce.price.is_a?(Float).should be_true
|
|
end
|
|
|
|
it "should return deterministic results when seeded" do
|
|
Faker.seed 123456
|
|
Faker::Commerce.color.should eq "salmon"
|
|
Faker::Commerce.department.should eq "Home, Movies & Computers"
|
|
Faker::Commerce.department(5).should eq "Toys & Home"
|
|
Faker::Commerce.department(2, true).should eq "Electronics & Tools"
|
|
Faker::Commerce.product_name.should eq "Heavy Duty Copper Lamp"
|
|
Faker::Commerce.price.should eq 47.25
|
|
Faker::Commerce.material.should eq "Rubber"
|
|
end
|
|
end
|