mirror of
https://github.com/niklasvh/html2canvas.git
synced 2023-08-10 21:13:10 +03:00
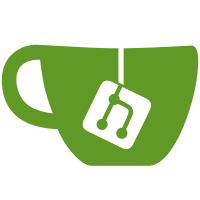
* initial typescript conversion * test: update overflow+transform ref test * fix: correctly render pseudo element content * fix: testrunner build * fix: karma test urls * test: update underline tests with <u> elements * test: update to es6-promise polyfill * test: remove watch from server * test: remove flow * format: update prettier for typescript * test: update eslint to use typescript parser * test: update linear gradient reftest * test: update test runner * test: update testrunner promise polyfill * fix: handle display: -webkit-flex correctly (fix #1817) * fix: correctly render gradients with clip & repeat (fix #1773) * fix: webkit-gradient function support * fix: implement radial gradients * fix: text-decoration rendering * fix: missing scroll positions for elements * ci: fix ios 11 tests * fix: ie logging * ci: improve device availability logging * fix: lint errors * ci: update to ios 12 * fix: check for console availability * ci: fix build dependency * test: update text reftests * fix: window reference for unit tests * feat: add hsl/hsla color support * fix: render options * fix: CSSKeyframesRule cssText Permission Denied on Internet Explorer 11 (#1830) * fix: option lint * fix: list type rendering * test: fix platform import * fix: ie css parsing for numbers * ci: add minified build * fix: form element rendering * fix: iframe rendering * fix: re-introduce experimental foreignobject renderer * fix: text-shadow rendering * feat: improve logging * fix: unit test logging * fix: cleanup resources * test: update overflow scrolling to work with ie * build: update build to include typings * fix: do not parse select element children * test: fix onclone test to work with older IEs * test: reduce reftest canvas sizes * test: remove dynamic setUp from list tests * test: update linear-gradient tests * build: remove old source files * build: update docs dependencies * build: fix typescript definition path * ci: include test.js on docs website
116 lines
4.4 KiB
TypeScript
116 lines
4.4 KiB
TypeScript
import {testList, ignoredTests} from '../build/reftests';
|
|
// @ts-ignore
|
|
import {default as platform} from 'platform';
|
|
// @ts-ignore
|
|
import Promise from 'es6-promise';
|
|
|
|
const testRunnerUrl = location.href;
|
|
const hasHistoryApi = typeof window.history !== 'undefined' && typeof window.history.replaceState !== 'undefined';
|
|
|
|
const uploadResults = (canvas: HTMLCanvasElement, url: string) => {
|
|
return new Promise((resolve: () => void, reject: (error: string) => void) => {
|
|
// @ts-ignore
|
|
const xhr = 'withCredentials' in new XMLHttpRequest() ? new XMLHttpRequest() : new XDomainRequest();
|
|
|
|
xhr.onload = () => {
|
|
if (typeof xhr.status !== 'number' || xhr.status === 200) {
|
|
resolve();
|
|
} else {
|
|
reject(`Failed to send screenshot with status ${xhr.status}`);
|
|
}
|
|
};
|
|
xhr.onerror = reject;
|
|
|
|
xhr.open('POST', 'http://localhost:8000/screenshot', true);
|
|
xhr.send(
|
|
JSON.stringify({
|
|
screenshot: canvas.toDataURL(),
|
|
test: url,
|
|
platform: {
|
|
name: platform.name,
|
|
version: platform.version
|
|
},
|
|
devicePixelRatio: window.devicePixelRatio || 1,
|
|
windowWidth: window.innerWidth,
|
|
windowHeight: window.innerHeight
|
|
})
|
|
);
|
|
});
|
|
};
|
|
|
|
testList
|
|
.filter(test => {
|
|
return !Array.isArray(ignoredTests[test]) || ignoredTests[test].indexOf(platform.name || '') === -1;
|
|
})
|
|
.forEach(url => {
|
|
describe(url, function() {
|
|
this.timeout(60000);
|
|
this.retries(2);
|
|
const windowWidth = 800;
|
|
const windowHeight = 600;
|
|
const testContainer = document.createElement('iframe');
|
|
testContainer.width = windowWidth.toString();
|
|
testContainer.height = windowHeight.toString();
|
|
testContainer.style.visibility = 'hidden';
|
|
testContainer.style.position = 'fixed';
|
|
testContainer.style.left = '10000px';
|
|
|
|
before(done => {
|
|
testContainer.onload = () => done();
|
|
|
|
testContainer.src = url + '?selenium&run=false&reftest&' + Math.random();
|
|
if (hasHistoryApi) {
|
|
// Chrome does not resolve relative background urls correctly inside of a nested iframe
|
|
try {
|
|
history.replaceState(null, '', url);
|
|
} catch (e) {}
|
|
}
|
|
|
|
document.body.appendChild(testContainer);
|
|
});
|
|
after(() => {
|
|
if (hasHistoryApi) {
|
|
try {
|
|
history.replaceState(null, '', testRunnerUrl);
|
|
} catch (e) {}
|
|
}
|
|
document.body.removeChild(testContainer);
|
|
});
|
|
it('Should render untainted canvas', () => {
|
|
const contentWindow = testContainer.contentWindow;
|
|
if (!contentWindow) {
|
|
throw new Error('Window not found for iframe');
|
|
}
|
|
|
|
return (
|
|
contentWindow
|
|
// @ts-ignore
|
|
.html2canvas(contentWindow.forceElement || contentWindow.document.documentElement, {
|
|
removeContainer: true,
|
|
backgroundColor: '#ffffff',
|
|
proxy: 'http://localhost:8081/proxy',
|
|
// @ts-ignore
|
|
...(contentWindow.h2cOptions || {})
|
|
})
|
|
.then((canvas: HTMLCanvasElement) => {
|
|
try {
|
|
(canvas.getContext('2d') as CanvasRenderingContext2D).getImageData(
|
|
0,
|
|
0,
|
|
canvas.width,
|
|
canvas.height
|
|
);
|
|
} catch (e) {
|
|
return Promise.reject('Canvas is tainted');
|
|
}
|
|
|
|
// @ts-ignore
|
|
if (window.__karma__) {
|
|
return uploadResults(canvas, url);
|
|
}
|
|
})
|
|
);
|
|
});
|
|
});
|
|
});
|