mirror of
https://github.com/piskelapp/piskel.git
synced 2023-08-10 21:12:52 +03:00
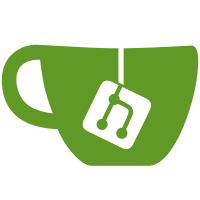
+ added explanatory comment for CanvasUtils.disableImageSmoothing + detect browser for chosing wheel/mousewheel event in DrawingController + create ABSTRACT_FUNCTION constant to be reused forabstract methods
49 lines
1.6 KiB
JavaScript
49 lines
1.6 KiB
JavaScript
(function () {
|
|
var ns = $.namespace("pskl");
|
|
|
|
ns.CanvasUtils = {
|
|
createCanvas : function (width, height, classList) {
|
|
var canvas = document.createElement("canvas");
|
|
canvas.setAttribute("width", width);
|
|
canvas.setAttribute("height", height);
|
|
|
|
if (typeof classList == "string") {
|
|
classList = [classList];
|
|
}
|
|
if (Array.isArray(classList)) {
|
|
for (var i = 0 ; i < classList.length ; i++) {
|
|
canvas.classList.add(classList[i]);
|
|
}
|
|
}
|
|
|
|
return canvas;
|
|
},
|
|
|
|
/**
|
|
* By default, all scaling operations on a Canvas 2D Context are performed using antialiasing.
|
|
* Resizing a 32x32 image to 320x320 will lead to a blurry output.
|
|
* On Chrome, FF and IE>=11, this can be disabled by setting a property on the Canvas 2D Context.
|
|
* In this case the browser will use a nearest-neighbor scaling.
|
|
* @param {Canvas} canvas
|
|
*/
|
|
disableImageSmoothing : function (canvas) {
|
|
var context = canvas.getContext('2d');
|
|
context.imageSmoothingEnabled = false;
|
|
context.mozImageSmoothingEnabled = false;
|
|
context.oImageSmoothingEnabled = false;
|
|
context.webkitImageSmoothingEnabled = false;
|
|
context.msImageSmoothingEnabled = false;
|
|
},
|
|
|
|
clear : function (canvas) {
|
|
if (canvas) {
|
|
canvas.getContext("2d").clearRect(0, 0, canvas.width, canvas.height);
|
|
}
|
|
},
|
|
|
|
getImageDataFromCanvas : function (canvas) {
|
|
var sourceContext = canvas.getContext('2d');
|
|
return sourceContext.getImageData(0, 0, canvas.width, canvas.height).data;
|
|
}
|
|
};
|
|
})(); |