mirror of
https://github.com/piskelapp/piskel.git
synced 2023-08-10 21:12:52 +03:00
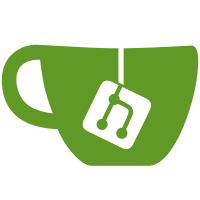
First commit : Removed Local storage feature Added 'download project' 'open project' options First attempt at simplifying right panel. To be continued ...
43 lines
1.4 KiB
JavaScript
43 lines
1.4 KiB
JavaScript
(function () {
|
|
var ns = $.namespace('pskl.utils');
|
|
|
|
var BASE64_REGEX = /\s*;\s*base64\s*(?:;|$)/i;
|
|
|
|
ns.BlobUtils = {
|
|
dataToBlob : function(dataURI, type, callback) {
|
|
var header_end = dataURI.indexOf(","),
|
|
data = dataURI.substring(header_end + 1),
|
|
isBase64 = BASE64_REGEX.test(dataURI.substring(0, header_end)),
|
|
blob;
|
|
|
|
if (Blob.fake) {
|
|
// no reason to decode a data: URI that's just going to become a data URI again
|
|
blob = new Blob();
|
|
blob.encoding = isBase64 ? "base64" : "URI";
|
|
blob.data = data;
|
|
blob.size = data.length;
|
|
} else if (Uint8Array) {
|
|
var blobData = isBase64 ? pskl.utils.Base64.decode(data) : decodeURIComponent(data);
|
|
blob = new Blob([blobData], {type: type});
|
|
}
|
|
callback(blob);
|
|
},
|
|
|
|
canvasToBlob : function(canvas, callback, type /*, ...args*/) {
|
|
type = type || "image/png";
|
|
|
|
if (canvas.mozGetAsFile) {
|
|
callback(canvas.mozGetAsFile("canvas", type));
|
|
} else {
|
|
var args = Array.prototype.slice.call(arguments, 2);
|
|
var dataURI = canvas.toDataURL.apply(canvas, args);
|
|
pskl.utils.BlobUtils.dataToBlob(dataURI, type, callback);
|
|
}
|
|
},
|
|
|
|
stringToBlob : function (string, callback, type) {
|
|
type = type || "text/plain";
|
|
pskl.utils.BlobUtils.dataToBlob('data:'+type+',' + string, type, callback);
|
|
}
|
|
};
|
|
})(); |