mirror of
https://github.com/lospec/pixel-editor.git
synced 2023-08-10 21:12:51 +03:00
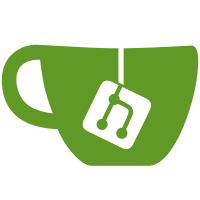
- added `/:paletteSlug/:resolution` functionality for localhost testing - created `currFile.sublayers` for *things that should zoom with the canvas layers* - `currFile.layers` now solely contains the canvas layers - added `getProjectData` to `FileManager`'s exported methods --- - added `FileManager.localStorageSave` (it's basically just: localStorage.setItem("lpe-cache",FileManager.getProjectData())) - added `FileManager.localStorageCheck` (it's basically just: `!!localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageLoad` (it's basically just: `return localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageReset` (for debugging purity) --- - calling `FileManager.localStorageSave()` on mouse up (we should stress test this) --- - changed lpe file format to `{canvasWidth:number,canvasHeight:number,selectedLayer:number,colors:[],layers:[]}` - added backward compatibility for the old lpe file format --- - added some canvas utility functions in `canvas_util` - added Unsettled's color similarity utility functions in `color_util2` --- - html boilerplate - wang tiles - - POC - tiny text boilerplate - POC - tiny text font scraper --- - WIP - added two optional url route parameters `/:paletteSlug/:resolution/:prefillWidth/:prefillBinaryStr` - WIP POC - hbs_parser.js (outputs tree data about hbs file relationships)
60 lines
2.0 KiB
JavaScript
60 lines
2.0 KiB
JavaScript
const PresetModule = (() => {
|
|
const presets = {
|
|
'Gameboy Color': {width: 240, height: 203, palette: 'Gameboy Color'},
|
|
'PICO-8': {width: 128, height: 128, palette: 'PICO-8'},
|
|
'Commodore 64': {width: 40, height: 80, palette: 'Commodore 64'}
|
|
};
|
|
|
|
function instrumentPresetMenu() {
|
|
//console.info("Initializing presets..");
|
|
// Add a button for all the presets available
|
|
const presetsMenu = document.getElementById('preset-menu');
|
|
Object.keys(presets).forEach((presetName,) => {
|
|
|
|
const button = document.createElement('button');
|
|
button.appendChild(document.createTextNode(presetName));
|
|
|
|
presetsMenu.appendChild(button);
|
|
|
|
button.addEventListener('click', () => {
|
|
////console.log("Preset: " + presetName);
|
|
//change dimentions on new pixel form
|
|
Util.setValue('size-width', presets[presetName].width);
|
|
Util.setValue('size-height', presets[presetName].height);
|
|
|
|
//set the text of the dropdown to the newly selected preset
|
|
Util.setText('palette-button', presets[presetName].palette);
|
|
|
|
//hide the dropdown menu
|
|
Util.deselect('preset-menu');
|
|
Util.deselect('preset-button');
|
|
|
|
//set the text of the dropdown to the newly selected preset
|
|
Util.setText('preset-button', presetName);
|
|
});
|
|
});
|
|
|
|
const presetButton = document.getElementById('preset-button');
|
|
presetButton.addEventListener('click', (e) => {
|
|
//open or close the preset menu
|
|
Util.toggle('preset-button');
|
|
Util.toggle('preset-menu');
|
|
|
|
//close the palette menu
|
|
Util.deselect('palette-button');
|
|
Util.deselect('palette-menu');
|
|
|
|
e.stopPropagation();
|
|
});
|
|
}
|
|
|
|
function propertiesOf(presetId) {
|
|
return presets[presetId];
|
|
}
|
|
|
|
return {
|
|
propertiesOf,
|
|
instrumentPresetMenu
|
|
};
|
|
|
|
})(); |