mirror of
https://github.com/lospec/pixel-editor.git
synced 2023-08-10 21:12:51 +03:00
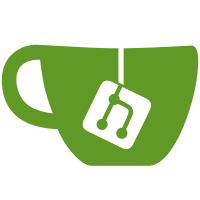
- added `/:paletteSlug/:resolution` functionality for localhost testing - created `currFile.sublayers` for *things that should zoom with the canvas layers* - `currFile.layers` now solely contains the canvas layers - added `getProjectData` to `FileManager`'s exported methods --- - added `FileManager.localStorageSave` (it's basically just: localStorage.setItem("lpe-cache",FileManager.getProjectData())) - added `FileManager.localStorageCheck` (it's basically just: `!!localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageLoad` (it's basically just: `return localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageReset` (for debugging purity) --- - calling `FileManager.localStorageSave()` on mouse up (we should stress test this) --- - changed lpe file format to `{canvasWidth:number,canvasHeight:number,selectedLayer:number,colors:[],layers:[]}` - added backward compatibility for the old lpe file format --- - added some canvas utility functions in `canvas_util` - added Unsettled's color similarity utility functions in `color_util2` --- - html boilerplate - wang tiles - - POC - tiny text boilerplate - POC - tiny text font scraper --- - WIP - added two optional url route parameters `/:paletteSlug/:resolution/:prefillWidth/:prefillBinaryStr` - WIP POC - hbs_parser.js (outputs tree data about hbs file relationships)
139 lines
5.2 KiB
JavaScript
139 lines
5.2 KiB
JavaScript
const TopMenuModule = (() => {
|
|
|
|
const mainMenuItems = document.getElementById('main-menu')?.children ?? [];
|
|
let infoList = document.getElementById('editor-info') ?? document.createElement("div");
|
|
let infoElements = {};
|
|
|
|
initMenu();
|
|
|
|
function initMenu() {
|
|
// for each button in main menu (starting at 1 to avoid logo), ending at length-1 to avoid
|
|
// editor info
|
|
for (let i = 1; i < mainMenuItems.length-1; i++) {
|
|
|
|
//get the button that's in the list item
|
|
const menuItem = mainMenuItems[i];
|
|
const menuButton = menuItem.children[0];
|
|
|
|
//when you click a main menu items button
|
|
Events.on('click', menuButton, function (e) {
|
|
// Close the already open menus
|
|
closeMenu();
|
|
// Select the item
|
|
Util.select(e.target.parentElement);
|
|
e.stopPropagation();
|
|
});
|
|
|
|
const subMenu = menuItem.children[1];
|
|
const subMenuItems = subMenu.children;
|
|
|
|
//when you click an item within a menu button
|
|
for (var j = 0; j < subMenuItems.length; j++) {
|
|
|
|
const currSubmenuItem = subMenuItems[j];
|
|
const currSubmenuButton = currSubmenuItem.children[0];
|
|
|
|
switch (currSubmenuButton.textContent) {
|
|
case 'New':
|
|
Events.on('click', currSubmenuButton, Dialogue.showDialogue, 'new-pixel');
|
|
break;
|
|
case 'Save project':
|
|
Events.on('click', currSubmenuButton, FileManager.openSaveProjectWindow);
|
|
break;
|
|
case 'Open':
|
|
Events.on('click', currSubmenuButton, FileManager.open);
|
|
break;
|
|
case 'Export':
|
|
Events.on('click', currSubmenuButton, FileManager.openPixelExportWindow);
|
|
break;
|
|
case 'Exit':
|
|
//if a document exists, make sure they want to delete it
|
|
if (EditorState.documentCreated()) {
|
|
//ask user if they want to leave
|
|
if (confirm('Exiting will discard your current pixel. Are you sure you want to do that?'))
|
|
//skip onbeforeunload prompt
|
|
window.onbeforeunload = null;
|
|
else
|
|
e.preventDefault();
|
|
}
|
|
break;
|
|
case 'Paste':
|
|
Events.on('click', currSubmenuButton, function(e){
|
|
Events.emit("ctrl+v");
|
|
e.stopPropagation();
|
|
});
|
|
break;
|
|
case 'Copy':
|
|
Events.on('click', currSubmenuButton, function(e){
|
|
Events.emit("ctrl+c");
|
|
e.stopPropagation();
|
|
});
|
|
break;
|
|
case 'Cut':
|
|
Events.on('click', currSubmenuButton, function(e){
|
|
Events.emit("ctrl+x");
|
|
e.stopPropagation();
|
|
});
|
|
break;
|
|
case 'Cancel':
|
|
Events.on('click', currSubmenuButton, function(e){
|
|
Events.emit("esc-pressed");
|
|
e.stopPropagation();
|
|
});
|
|
break;
|
|
//Help Menu
|
|
case 'Settings':
|
|
Events.on('click', currSubmenuButton, Dialogue.showDialogue, 'settings');
|
|
break;
|
|
case 'Help':
|
|
Events.on('click', currSubmenuButton, Dialogue.showDialogue, 'help');
|
|
break;
|
|
case 'About':
|
|
Events.on('click', currSubmenuButton, Dialogue.showDialogue, 'about');
|
|
break;
|
|
case 'Changelog':
|
|
Events.on('click', currSubmenuButton, Dialogue.showDialogue, 'changelog');
|
|
break;
|
|
}
|
|
|
|
Events.on('click', currSubmenuButton, function() {TopMenuModule.closeMenu();});
|
|
}
|
|
}
|
|
}
|
|
|
|
function closeMenu () {
|
|
//remove .selected class from all menu buttons
|
|
for (var i = 0; i < mainMenuItems.length; i++) {
|
|
Util.deselect(mainMenuItems[i]);
|
|
}
|
|
}
|
|
|
|
function resetInfos() {
|
|
infoList.innerHTML = "<ul></ul>";
|
|
}
|
|
|
|
function updateField(fieldId, value) {
|
|
const elm = document.getElementById(fieldId);
|
|
if(elm) {
|
|
elm.value = value;
|
|
} else {
|
|
//console.warn('elm === ', elm);
|
|
}
|
|
}
|
|
|
|
function addInfoElement(fieldId, field) {
|
|
let liEl = document.createElement("li");
|
|
|
|
infoElements[fieldId] = field;
|
|
liEl.appendChild(field);
|
|
|
|
infoList.firstChild.appendChild(liEl);
|
|
}
|
|
|
|
return {
|
|
closeMenu,
|
|
updateField,
|
|
addInfoElement,
|
|
resetInfos
|
|
}
|
|
})(); |