mirror of
https://github.com/lospec/pixel-editor.git
synced 2023-08-10 21:12:51 +03:00
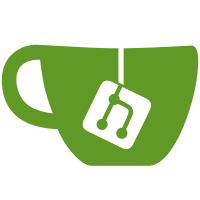
- added `/:paletteSlug/:resolution` functionality for localhost testing - created `currFile.sublayers` for *things that should zoom with the canvas layers* - `currFile.layers` now solely contains the canvas layers - added `getProjectData` to `FileManager`'s exported methods --- - added `FileManager.localStorageSave` (it's basically just: localStorage.setItem("lpe-cache",FileManager.getProjectData())) - added `FileManager.localStorageCheck` (it's basically just: `!!localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageLoad` (it's basically just: `return localStorage.getItem("lpe-cache")`) - added `FileManager.localStorageReset` (for debugging purity) --- - calling `FileManager.localStorageSave()` on mouse up (we should stress test this) --- - changed lpe file format to `{canvasWidth:number,canvasHeight:number,selectedLayer:number,colors:[],layers:[]}` - added backward compatibility for the old lpe file format --- - added some canvas utility functions in `canvas_util` - added Unsettled's color similarity utility functions in `color_util2` --- - html boilerplate - wang tiles - - POC - tiny text boilerplate - POC - tiny text font scraper --- - WIP - added two optional url route parameters `/:paletteSlug/:resolution/:prefillWidth/:prefillBinaryStr` - WIP POC - hbs_parser.js (outputs tree data about hbs file relationships)
89 lines
2.6 KiB
JavaScript
89 lines
2.6 KiB
JavaScript
/** Handles the pop up windows (NewPixel, ResizeCanvas ecc)
|
|
*
|
|
*/
|
|
const Dialogue = (() => {
|
|
let currentOpenDialogue = "";
|
|
let dialogueOpen = true;
|
|
|
|
const popUpContainer = document.getElementById("pop-up-container") ?? document.createElement("div");
|
|
const cancelButtons = popUpContainer.getElementsByClassName('close-button');
|
|
|
|
Events.onCustom("esc-pressed", closeDialogue);
|
|
|
|
// Add click handlers for all cancel buttons
|
|
for (var i = 0; i < cancelButtons.length; i++) {
|
|
cancelButtons[i].addEventListener('click', function () {
|
|
closeDialogue();
|
|
});
|
|
}
|
|
|
|
/** Closes a dialogue window if the user clicks everywhere but in the current window
|
|
*
|
|
*/
|
|
popUpContainer.addEventListener('click', function (e) {
|
|
if (e.target == popUpContainer)
|
|
closeDialogue();
|
|
});
|
|
|
|
/** Shows the dialogue window called dialogueName, which is a child of pop-up-container in pixel-editor.hbs
|
|
*
|
|
* @param {*} dialogueName The name of the window to show
|
|
* @param {*} trackEvent Should I track the GA event?
|
|
*/
|
|
function showDialogue (dialogueName, trackEvent) {
|
|
|
|
|
|
|
|
if (typeof trackEvent === 'undefined') trackEvent = true;
|
|
|
|
// Updating currently open dialogue
|
|
currentOpenDialogue = dialogueName;
|
|
// The pop up window is open
|
|
dialogueOpen = true;
|
|
// Showing the pop up container
|
|
popUpContainer.style.display = 'block';
|
|
|
|
// Showing the window
|
|
document.getElementById(dialogueName).style.display = 'block';
|
|
|
|
// If I'm opening the palette window, I initialize the colour picker
|
|
if (dialogueName == 'palette-block' && EditorState.documentCreated()) {
|
|
ColorPicker.init();
|
|
PaletteBlock.init();
|
|
}
|
|
|
|
//track google event
|
|
if (trackEvent && typeof ga !== 'undefined')
|
|
ga('send', 'event', 'Palette Editor Dialogue', dialogueName); /*global ga*/
|
|
}
|
|
|
|
/** Closes the current dialogue by hiding the window and the pop-up-container
|
|
*
|
|
*/
|
|
function closeDialogue () {
|
|
popUpContainer.style.display = 'none';
|
|
var popups = popUpContainer.children;
|
|
|
|
for (var i = 0; i < popups.length; i++) {
|
|
popups[i].style.display = 'none';
|
|
}
|
|
|
|
dialogueOpen = false;
|
|
|
|
if (currentOpenDialogue == "palette-block") {
|
|
ColorModule.addToSimplePalette();
|
|
}
|
|
}
|
|
|
|
function isOpen() {
|
|
return dialogueOpen;
|
|
}
|
|
|
|
return {
|
|
showDialogue,
|
|
closeDialogue,
|
|
isOpen
|
|
}
|
|
})();
|
|
|
|
////console.log("Dialog: " + Dialogue);
|