mirror of
https://github.com/eternnoir/pyTelegramBotAPI.git
synced 2023-08-10 21:12:57 +03:00
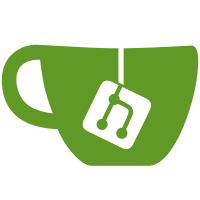
Fixed InlineQueryResultVideo params in example. String "Video" was passed in stead of the thumbnail url, making the example not work, This has been tested working.
75 lines
2.8 KiB
Python
75 lines
2.8 KiB
Python
# This example show how to write an inline mode telegram bot use pyTelegramBotAPI.
|
|
import logging
|
|
import sys
|
|
import time
|
|
|
|
import telebot
|
|
from telebot import types
|
|
|
|
API_TOKEN = '<TOKEN>'
|
|
|
|
bot = telebot.TeleBot(API_TOKEN)
|
|
telebot.logger.setLevel(logging.DEBUG)
|
|
|
|
|
|
@bot.inline_handler(lambda query: query.query == 'text')
|
|
def query_text(inline_query):
|
|
try:
|
|
r = types.InlineQueryResultArticle('1', 'Result1', types.InputTextMessageContent('hi'))
|
|
r2 = types.InlineQueryResultArticle('2', 'Result2', types.InputTextMessageContent('hi'))
|
|
bot.answer_inline_query(inline_query.id, [r, r2])
|
|
except Exception as e:
|
|
print(e)
|
|
|
|
|
|
@bot.inline_handler(lambda query: query.query == 'photo1')
|
|
def query_photo(inline_query):
|
|
try:
|
|
r = types.InlineQueryResultPhoto('1',
|
|
'https://raw.githubusercontent.com/eternnoir/pyTelegramBotAPI/master/examples/detailed_example/kitten.jpg',
|
|
'https://raw.githubusercontent.com/eternnoir/pyTelegramBotAPI/master/examples/detailed_example/kitten.jpg',
|
|
input_message_content=types.InputTextMessageContent('hi'))
|
|
r2 = types.InlineQueryResultPhoto('2',
|
|
'https://raw.githubusercontent.com/eternnoir/pyTelegramBotAPI/master/examples/detailed_example/rooster.jpg',
|
|
'https://raw.githubusercontent.com/eternnoir/pyTelegramBotAPI/master/examples/detailed_example/rooster.jpg')
|
|
bot.answer_inline_query(inline_query.id, [r, r2], cache_time=1)
|
|
except Exception as e:
|
|
print(e)
|
|
|
|
|
|
@bot.inline_handler(lambda query: query.query == 'video')
|
|
def query_video(inline_query):
|
|
try:
|
|
r = types.InlineQueryResultVideo('1',
|
|
'https://github.com/eternnoir/pyTelegramBotAPI/blob/master/tests/test_data/test_video.mp4?raw=true',
|
|
'video/mp4',
|
|
'https://raw.githubusercontent.com/eternnoir/pyTelegramBotAPI/master/examples/detailed_example/rooster.jpg',
|
|
'Title'
|
|
)
|
|
bot.answer_inline_query(inline_query.id, [r])
|
|
except Exception as e:
|
|
print(e)
|
|
|
|
|
|
@bot.inline_handler(lambda query: len(query.query) == 0)
|
|
def default_query(inline_query):
|
|
try:
|
|
r = types.InlineQueryResultArticle('1', 'default', types.InputTextMessageContent('default'))
|
|
bot.answer_inline_query(inline_query.id, [r])
|
|
except Exception as e:
|
|
print(e)
|
|
|
|
|
|
def main_loop():
|
|
bot.infinity_polling()
|
|
while 1:
|
|
time.sleep(3)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
try:
|
|
main_loop()
|
|
except KeyboardInterrupt:
|
|
print('\nExiting by user request.\n')
|
|
sys.exit(0)
|