mirror of
https://github.com/vlang/v.git
synced 2023-08-10 21:13:21 +03:00
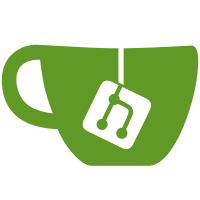
* feat: json2 time.Time encode
* refactor: best var name
* fix: use 'RFC 3339' as pattern in json encode
* fix: unused var for unaliased_typ
* fix: improve test
* use the clearer test after V ad24c22
, that made format_rfc3339 more stable
Co-authored-by: Delyan Angelov <delian66@gmail.com>
47 lines
961 B
V
47 lines
961 B
V
import x.json2 as json
|
|
|
|
enum JobTitle {
|
|
manager
|
|
executive
|
|
worker
|
|
}
|
|
|
|
struct Employee {
|
|
pub mut:
|
|
name string
|
|
age int
|
|
salary f32
|
|
title JobTitle
|
|
}
|
|
|
|
fn test_fast_raw_decode() {
|
|
s := '{"name":"Peter","age":28,"salary":95000.5,"title":2}'
|
|
o := json.fast_raw_decode(s) or {
|
|
assert false
|
|
json.Any(json.null)
|
|
}
|
|
str := o.str()
|
|
assert str == '{"name":"Peter","age":"28","salary":"95000.5","title":"2"}'
|
|
}
|
|
|
|
fn test_character_unescape() {
|
|
message := r'{
|
|
"newline": "new\nline",
|
|
"tab": "\ttab",
|
|
"backslash": "back\\slash",
|
|
"quotes": "\"quotes\"",
|
|
"slash":"\/dev\/null"
|
|
}'
|
|
mut obj := json.raw_decode(message) or {
|
|
println(err)
|
|
assert false
|
|
return
|
|
}
|
|
lines := obj.as_map()
|
|
assert lines['newline'] or { 0 }.str() == 'new\nline'
|
|
assert lines['tab'] or { 0 }.str() == '\ttab'
|
|
assert lines['backslash'] or { 0 }.str() == 'back\\slash'
|
|
assert lines['quotes'] or { 0 }.str() == '"quotes"'
|
|
assert lines['slash'] or { 0 }.str() == '/dev/null'
|
|
}
|