mirror of
https://github.com/vlang/v.git
synced 2023-08-10 21:13:21 +03:00
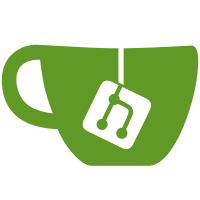
* termio: new termio module move the tcgetattr and tcsetattr functions in a new termio module. The code needed refactoring as different OS have different fields size, position and number for the C.termios structure, which could not be correctly expressed consitently otherwise. It has the positive side effect to reduce the number of unsafe calls. New testing code was also added for the readline module as it is relying of the feature. * apply 2023 copyright to the new files too
37 lines
1015 B
V
37 lines
1015 B
V
// Copyright (c) 2019-2023 Alexander Medvednikov. All rights reserved.
|
|
// Use of this source code is governed by an MIT license
|
|
// that can be found in the LICENSE file.
|
|
//
|
|
// Serves as a more advanced input method
|
|
// based on the work of https://github.com/AmokHuginnsson/replxx
|
|
//
|
|
module readline
|
|
|
|
import term.termios
|
|
|
|
// Winsize stores the screen information on Linux.
|
|
struct Winsize {
|
|
ws_row u16
|
|
ws_col u16
|
|
ws_xpixel u16
|
|
ws_ypixel u16
|
|
}
|
|
|
|
// Readline is the key struct for reading and holding user input via a terminal.
|
|
// Example: import readline { Readline }
|
|
pub struct Readline {
|
|
mut:
|
|
is_raw bool
|
|
orig_termios termios.Termios // Linux
|
|
current []rune // Line being edited
|
|
cursor int // Cursor position
|
|
overwrite bool
|
|
cursor_row_offset int
|
|
prompt string
|
|
prompt_offset int
|
|
previous_lines [][]rune
|
|
skip_empty bool // skip the empty lines when calling .history_previous()
|
|
search_index int
|
|
is_tty bool
|
|
}
|